Inheritance, Samples of using inheritance
Inheritance is the property by which one object can
inherit the properties of the other object. A general class can be
inherited by the other classes. A class that is inherited is called a
base class. A class which is inheriting another class is called a
derived class. When a class inherits another class, members of the base
class become the members of the derived class. The general form of
inheritance is:-
class derived_name : access_specifier base_name
{
};
The derived_name is the name of the derived class.
The base_name is the name of the base class. The access_specifier can
be private, public or protected. If the access_specifier is public then
all public members of the base class become public members of the
derived class and protected members of the base class become the
protected members of the derived class. If the access_specifier is
private then all public and protected members of the base class will
become private members of the derived class. If the access_specifier is
protected then the public and protected members of the base class
become the protected members of the derived class. Whether
access_specifier is public, private or protected, private members of the
base class will not be accessed by the members of the derived class.
The access_specifier protected provides more
flexibility in terms of inheritance. The private members of the base
class cannot be accessed by the members of the derived class. The
protected members of the base class remain private to their class but
can be accessed and inherited by the derived class. The protected
members of the base class will remain private to the other elements of
the program.
A derived class can inherit one or more base
classes. A constructor of the base is executed first and then the
constructor of derived class is executed. A destructor of derived class
is called before the destructor of base class. The arguments to the
base class constructor can be passed as follows:-
derived_constructor (argument list): base1 (arg_list)
base2(arg_list1)
baseN(arg_list)
The derived_constructor is the name of the derived
class. The argument list is list of the data members of the derived
class. The base1 is name of the base class. The arg_list is the list of
the members of the base class. Here is a program which illustrates the
features of inheritance.
#include<iostream>
using namespace std;
class shape
{
private :
double length;
protected:
double breadth;
public :
double len()
{
return(length);
}
shape(double length1,double breadth1)
{
length=length1;
breadth=breadth1;
}
//shape() { }
};
class shape1
{
public:
double height;
shape1(double height1)
{
height=height1;
}
//shape1() { }
};
class cuboid : public shape, private shape1
{
public:
cuboid(double length1,double breadth1,double height1):shape(length1,breadth1),shape1(height1)
{
cout << " A constructor is called " << endl;
}
double volume()
{
return(height*breadth*len());
}
double bre()
{
return(breadth);
}
double ht()
{
return(height);
}
};
int main()
{
cuboid c1(2.4,3.5,6.7);
cout << "The length of the cuboid is : " << c1.len() << endl;
cout << "The breadth of the cuboid is : " << c1.bre() << endl;
cout << "The height of the cuboid is : " << c1.ht() << endl;
cout << "The volume of the cuboid is : " << c1.volume() << endl;
return(0);
}
The result of the program is:-
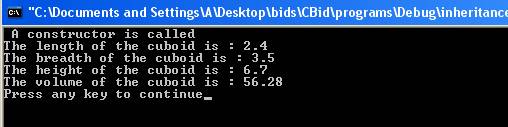
The program has two base classes shape and shape1
and one derived class called cuboid which inherits shape as public and
shape1 as private. The public and protected members of shape become
pubic and protected members of derived class cuboid. The private
members of shape remain private to the class shape. The members of
shape1 class become the private members of the derived class cuboid.
The statement
class cuboid : public shape, private shape1
states that class cuboid inherits class shape as public and class shape1 as private. The statement
cuboid(double length1,double breadth1,double height1):shape(length1,breadth1),shape1(height1)
{
cout << " A constructor is called " << endl;
}
declares the constructor of the class cuboid. When
constructor of class cuboid is called first constructor of shape is
executed and then constructor of shape1 is executed and after that the
constructor of cuboid is executed. The statements
double volume()
{
return(height*breadth*len());
}
calculate the volume of the cuboid. The class
cuboid cannot access the private data member length of the shape class.
It access the length by calling the function len() which returns the
private data member length. The data member breadth becomes the
protected member of the class cuboid. The height which is public member
of shape1 class becomes the private member of the class cuboid as it
inherits the shape 1 class as private. The statements
double bre()
{
return(breadth);
}
returns the breadth of the cuboid as data member
breadth cannot be accessed outside the class as it is protected member
of cuboid. The statement
double ht()
{
return(height);
}
returns the height of the cuboid as data member
height cannot be accessed outside the class as height is the private
data member of the class cuboid. The statement
cuboid c1(2.4,3.5,6.7);
creates an object c1 of type cuboid. The
constructor is called to initialize the values of the cuboid. The
constructor of shape is executed and then constructor of shape1 is
executed and then finally constructor of cuboid is executed. The
statement
cout << "The length of the cuboid is : " << c1.len() << endl;
displays the length of the cuboid as c1.len() calls
the len() function of class shape which is also the public member
function of cuboid. The statement
cout << "The breadth of the cuboid is : " << c1.bre() << endl;
displays the breadth of the cuboid. As the data
member breadth cannot be accessed directly as it is protected member of
the class cuboid so the function bre() returns the breadth of the
cuboid. The statement
cout << "The height of the cuboid is : " << c1.ht() << endl;
displays the height of the cuboid. The data member
height cannot be accessed directly as it is private member of class
cuboid so it is accessed through the function ht() which returns
height.