Class and Objects
Constructor and Destructor with Methods and properties
A class is a user defined data type like a
structure or a union. A class consists of data variables and functions.
These variables and functions are called members of the class. The
variables are called data members and functions are called member
functions. The member functions are also called methods. The data
members are called properties of the class. An object is the instance
of the class. An object is like a compound variable of the user defined
type. It links both code and data. Within the object, members of the
class can be public or private to the object. The declaration of a
class is syntactically same as structure. The class is declared using
keyword class. The general form of the declaration of the class is:-
class class_name
{
access_specifier:
data functions
access_specifier:
data functions
} object_list;
The object_list is optional. The object_list is
used to declare objects of the class. The class_name is the name of the
class. The access_specifier can either public, private or protected.
The members of the class by default are private to the class. If the
access_specifier is private then members of the class are not
accessible outside the class. If the access_specifier is public then
members of the class can be accessed from outside the class. The
protected access_specifier is needed at the time of inheritance. The
members can be accessed using an object’s name, a dot operator and name
of the member. Here is a program which shows how classes and objects
are created.
#include<iostream>
using namespace std;
class cube
{
public:
double side;
double volume()
{
return(side*side*side);
}
};
int main()
{
double volume1=0;
cube c1,c2;
cout << "Enter the lenght of the cube" << endl;
cin >> c1.side;
cout << "The volume of the cube is : " << c1.volume() << endl;
c2.side=c1.side +2;
cout << "The volume of the second cube is : " << c2.volume() << endl;
return(0);
}
The result of the program is:-

The program consists of a class cube which has data
member side of type double and member function which calculates the
volume of the cube. The statement
class cube
declares a class cube. The statements
public:
double side;
double volume()
{
return(side*side*side);
}
declare that access_specifier is public for data
member side and member function volume. These members can be accessed
from the other parts of the program. The statement
cube c1,c2;
declares two objects c1 and c2 of type cube. The statement
cin >> c1.side;
access the data member of the cube. The member is
accessed by specifying the name of the object as c1 then dot operator
and then name of the variable side. The length entered by the user is
stored in c1.side. In the statement
cout << "The volume of the cube is : " << c1.volume() << endl;
c1.volume() calls the member function volume which
returns the volume of the cube of side whose length is entered by the
user. The statement
c2.side=c1.side +2;
equates the side of object c2 to side of object c1 increased by 2. The objects c2 and c1 are different. The statement
cout << "The volume of the second cube is : " << c2.volume() << endl;
displays the volume of second object c2.
Constructor and Destructor:
Constructors are used in order to initialize the
objects. A constructor is a special kind of a function which is the
member of the class. The name of the constructor is same as name of the
class. A constructor is automatically called when object is created. A
constructor does not have a return type.
A default constructor is a constructor with no
parameters. If no constructor is defined by the user then compiler
supplies the default constructor. Once the constructor is defined by
the user then compiler does not supply default constructor and then
user is responsible for defining default constructor.
A destructor is the complement of the constructor.
It is used to destroy the objects. The objects are destroyed in order
to deallocate the memory occupied by them. The name of the destructor
is same as the name of the constructor as is preceded by a tilt
operator ‘~’. A destructor for objects is executed in the reverse order
of the constructor functions.
Here is a program which shows how constructors and destructors are used.
#include<iostream>
using namespace std;
class cube
{
public:
double side;
double volume()
{
return(side*side*side);
}
cube(double side1)
{
cout << "A constructor is called" << endl;
side=side1;
}
cube()
{
cout << "A default constructor is called " << endl;
}
~cube()
{
cout << "Destructing " << side << endl;
}
};
int main()
{
cube c1(2.34);
cube c2;
cout << "The side of the cube is: " << c1.side << endl;
cout << "The volume of the first cube is : " << c1.volume() << endl;
cout << "Enter the length of the second cube : " ;
cin >> c2.side;
cout << "The volume of second cube is : " << c2.volume() << endl;
return(0);
}
The result of the program is:-
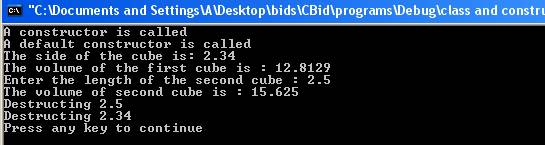
The statement
cube(double side1)
{
cout << "A constructor is called" << endl;
side=side1;
}
declares the constructor of the class cube. The
name of the constructor is same as the name of the class. There is no
return type in the constructor. It will initialize the value of data
member side. The statement
cube()
{
cout << "A default constructor is called " << endl;
}
declares a default constructor. The statement
~cube()
{
cout << "Destructing " << side << endl;
}
declares a destructor to deallocate the objects. The statement
cube c1(2.34);
creates an object c1 of type cube. A constructor is
automatically called and initializes the data member side with value
2.34. The statement
cube c2;
creates an object of type c2. When object c2 is created a default constructor is called and the message will be printed. The statements
cout << "The side of the cube is: " << c1.side << endl;
cout << "The volume of the first cube is : " << c1.volume() << endl;
displays the side and volume of the cube where side has value 2.34. The statement
cin >> c2.side;
will set the value of
the side of the object c2 as entered by the user. At the end of the
program objects are deallocated in the reverse order in which
constructors are called. First object c2 is deallocated whose side is
2.5 and then object c1 is deallocated whose side is 2.34.
Advantage of the classes:-
It provides protection to the data. The members of
the class are by default private to the class while the members of the
structure are public. OOP features allow programmer to easily handle
complex problems and multi file projects. They help in modeling real
world objects such as bank accounts and their related transactions.
Exercise-1: One steps through integer points of the straight line. The length of a step must be non negative and can be by one bigger than, equal to, or by one smaller than the length of the previous step. What is the minimum number of steps in order to get from x to y ? The length of the f irst and the last step must be 1. View Details
Exercise-2: Some operators checks about the relationship between two values and these operators are called relational operators.
Given two numerical values your job is just to nd out the relationship between them that is
(i) First one is greater than the second
(ii) First one is less than the second or
(iii) First and second one is equal.
View Details
Exercise-1: One steps through integer points of the straight line. The length of a step must be non negative and can be by one bigger than, equal to, or by one smaller than the length of the previous step. What is the minimum number of steps in order to get from x to y ? The length of the f irst and the last step must be 1. View Details
Exercise-2: Some operators checks about the relationship between two values and these operators are called relational operators.
Given two numerical values your job is just to nd out the relationship between them that is
(i) First one is greater than the second
(ii) First one is less than the second or
(iii) First and second one is equal.
View Details
45th CSE
ReplyDeleteCSE 45th
ReplyDeleteusing C++
ReplyDeleteid -- 201510121
#include
using namespace std;
class Box
{
public:
double width;
double length;
double height;
Box();
Box(double width, double height, double length);
~Box();
};
Box::Box()
{
cout << "It will be calculate the area of box.\n";
cout << "Enter the value of width of your box --: ";
cin >> width;
cout << "Enter the value of length of your box --: ";
cin >> length;
cout << "Enter the value of height of your box --: ";
cin >> height;
cout << "The area of the box is --: " << width*height*length << "\n";
}
Box::Box(double width, double height, double length)
{
cout << "The area of the box is --: " << width*height*length << "\n";
}
Box::~Box()
{
cout << "[+]Memory released[+]";
}
int main()
{
Box objOne;
double width;
double length;
double height;
cout << "This will be also calculate the area of box -- \n";
cout << "Enter the value of width of your box --: ";
cin >> width;
cout << "Enter the value of length of your box --: ";
cin >> length;
cout << "Enter the value of height of your box --: ";
cin >> height;
Box ObjTwo(width, height, length);
return 0;
}
using c++
ReplyDeleteid -- 201510121
#include
using namespace std;
class Box
{
public:
double width;
double length;
double height;
Box();
Box(double width, double height, double length);
~Box();
};
Box::Box()
{
cout << "It will be calculate the area of box.\n";
cout << "Enter the value of width of your box --: ";
cin >> width;
cout << "Enter the value of length of your box --: ";
cin >> length;
cout << "Enter the value of height of your box --: ";
cin >> height;
cout << "The area of the box is --: " << width*height*length << "\n";
}
Box::Box(double width, double height, double length)
{
cout << "The area of the box is --: " << width*height*length << "\n";
}
Box::~Box()
{
cout << "[+]Memory released[+]";
}
int main()
{
Box objOne;
double width;
double length;
double height;
cout << "This will be also calculate the area of box -- \n";
cout << "Enter the value of width of your box --: ";
cin >> width;
cout << "Enter the value of length of your box --: ";
cin >> length;
cout << "Enter the value of height of your box --: ";
cin >> height;
Box ObjTwo(width, height, length);
return 0;
}
Mahabub rahman
ReplyDelete#include
using namespace std;
class box
{
public:
double length;
double breadth;
double hight;
double volume()
{
return(length*breadth*hight);
}
box(double l,double b,double h)
{
cout << "A constructor is called" << endl;
length=l;
breadth=b;
hight=h;
}
box()
{
cout << "A default constructor is called " << endl;
}
~box()
{
cout << "Destructing " << length << breadth << hight << endl;
}
};
int main()
{
box b1(4.14,4.43,3.14);
box b2;
cout << "The Box length is: " << b1.length << endl;
cout << "The Box breadth is: " << b1.breadth << endl;
cout << "The Box hight is: " << b1.hight << endl;
cout << "The area of the first Box is : " << b1.volume() << endl;
cout << "Enter the length of the second Box : " ;
cin >> b2.length;
cout << "Enter the breadth of the second Box : " ;
cin >> b2.breadth;
cout << "Enter the hight of the second Box : " ;
cin >> b2.hight;
cout << "The area of second Box is : \n " << b2.volume() << endl;
return(0);
}
47th CS ID= 201430852
ReplyDelete#include
using namespace std;
class box
{
public:
double length;
double breadth;
double hight;
double volume()
{
return(length*breadth*hight);
}
box(double l,double b,double h)
{
cout << "A constructor is called" << endl;
length=l;
breadth=b;
hight=h;
}
box()
{
cout << "A default constructor is called " << endl;
}
~box()
{
cout << "Destructing " << length << breadth << hight << endl;
}
};
int main()
{
box b1(4.14,4.43,3.14);
box b2;
cout << "The Box length is: " << b1.length << endl;
cout << "The Box breadth is: " << b1.breadth << endl;
cout << "The Box hight is: " << b1.hight << endl;
cout << "The area of the first Box is : " << b1.volume() << endl;
cout << "Enter the length of the second Box : " ;
cin >> b2.length;
cout << "Enter the breadth of the second Box : " ;
cin >> b2.breadth;
cout << "Enter the hight of the second Box : " ;
cin >> b2.hight;
cout << "The area of second Box is : \n " << b2.volume() << endl;
return(0);
}
#include
ReplyDeleteusing namespace std;
class Box
{
public:
double length,height,breadth;
double volume()
{
return (length*height*breadth);
}
Box(double x,double y,double z)
{
length=x;
height=y;
breadth=z;
}
Box();
~Box()
{
cout <<"Destructing activate"<< endl;
}
};
int main()
{
double i, j, k;
cout <<"Enter the length of box1: ";
cin >> i;
cout<<"Enter the height of box1: ";
cin>>j;
cout<<"Enter the breadth of box1: ";
cin>>k;
Box box1(i,j,k);
cout<<"Enter the length of box2: ";
cin>>i;
cout<<"Enter the height of box2: ";
cin>>j;
cout<<"Enter the breadth of box2: ";
cin>>k;
Box box2(i,j,k);
cout <<"The volume of box1: "<< box1.volume() <<endl;
cout<<"The volume of box2: "<< box2.volume() <<endl;
return 0;
}
id: 201510660
problem 2
ReplyDeleteusing C++
#include
using namespace std;
class cons
{
public:
int testCase, r = 2;
cons(int tCase);
~cons();
void result();
};
cons::cons(int tCase)
{
testCase = tCase;
}
cons::~cons()
{
}
void cons::result()
{
int arr[testCase][r];
for (int i = 0; i < testCase; i++){
for (int j = 0; j < r; j++)
cin >> arr[i][j];
}
cout << "\n";
for (int i = 0; i < testCase; i++){
for (int j = 0; j < r-1; j++){
if (arr[i][j] > arr[i][j+1])
cout << ">\n";
else if (arr[i][j] < arr[i][j+1])
cout << "<\n";
else if (arr[i][j] == arr[i][j+1])
cout << "=\n";
}
}
}
int main()
{
int t;
cin >> t;
if (t < 15){
cons obj(t);
obj.result();
}
return 0;
}
problem 2:
ReplyDelete#include
using namespace std;
class relational_operator
{
public:
int testcase;
relational_operator();
~relational_operator();
void result();
};
relational_operator::relational_operator()
{
int i;
cout<<"Number of testcase: ";
cin>>testcase;
}
void relational_operator::result()
{
int i,a,b;
if(testcase<15)
{
for(i=1; i<=testcase; i++)
{
cout<>a>>b;
if(a<1000000001 && b<1000000001)
{
if(ab)
cout<<">"<<endl;
else
cout<<"="<<endl;
}
}
}
else
cout<<endl<<"SORRY, testcase doesn't match :) "<<endl;
}
relational_operator::~relational_operator()
{
}
main()
{
relational_operator ob;
ob.result();
}
problem 2;
ReplyDeleteid 201510469;
#include
using namespace std;
class rectangle
{
int height;
int width;
public:
void set(int h,int w);
int area();
rectangle();
~rectangle();
};
int rectangle::area()
{
return height*width;
}
rectangle::rectangle()
{
height=5;
width=6;
cout<<"constructor"<<endl;
}
rectangle::~rectangle()
{
cout<<"Destructor"<<endl;
}
int main()
{
rectangle obj;
cout<<"area="<<obj.area()<<endl;
return 0;
}
#include
ReplyDeleteusing namespace std;
class operate
{
public:
int test,i,j;
int range=2;
operate();
~operate()
{
cout<<"Distructor active";
}
};
operate::operate()
{
cout<<"Enter the array size: ";
cin>>test;
int arr[test][range];
for(int i=0;i>arr[i][j];
}
for (int i=0;iarr[i][j+1])
cout<<"Relational operator is: >"<<endl;
else if(arr[i][j]<arr[i][j+1])
cout<<"Relational operator is: <"<<endl;
else if(arr[i][j]==arr[i][j+1])
cout<<"Relational operator is: ="<<endl;
}
}
}
int main()
{
operate obj;
return 0;
}
id:201510660
#include
ReplyDeleteusing namespace std;
class operate
{
public:
int siz,i,j;
int ran=2;
operate();
~operate()
{
cout<<"Distructor active";
}
};
operate::operate()
{
cout<<"Enter the array size: ";
cin>>siz;
int arr[siz][ran];
for(int i=0;i>arr[i][j];
}
for (int i=0;iarr[i][j+1])
cout<<"Relational operator is: >"<<endl;
else if(arr[i][j]<arr[i][j+1])
cout<<"Relational operator is: <"<<endl;
else if(arr[i][j]==arr[i][j+1])
cout<<"Relational operator is: ="<<endl;
}
}
}
int main()
{
operate obj;
return 0;
}
id:201510992
#include
ReplyDeleteusing namespace std;
class find_op
{
public:
int x,y,n;
find_op();
void show();
~find_op();
};
find_op::find_op()
{
cout<<"Enter test case: ";
cin>>n;
cout<>x>>y;
cout<y)
cout<<">"<<endl<<endl;
else if(x<y)
cout<<"<"<<endl<<endl;
else
cout<<"="<<endl<<endl;
}
}
find_op::~find_op()
{
}
int main()
{
find_op op;
op.show();
return 0;
}
#include
ReplyDeleteusing namespace std;
class operate
{
public:
int test,i,j;
int range=2;
operate();
~operate()
{
cout<<"Distructor active";
}
};
operate::operate()
{
cout<<"Enter the array size: ";
cin>>test;
int arr[test][range];
for(int i=0;i>arr[i][j];
}
for (int i=0;iarr[i][j+1])
cout<<"Relational operator is: >"<<endl;
else if(arr[i][j]<arr[i][j+1])
cout<<"Relational operator is: <"<<endl;
else if(arr[i][j]==arr[i][j+1])
cout<<"Relational operator is: ="<<endl;
}
}
}
int main()
{
operate obj;
return 0;
}
PROBLEM 1:SOLUTION 1
ReplyDelete#include
//#include
using namespace std;
class straight_line
{
public:
int n;
straight_line();
void show();
};
straight_line::straight_line()
{
cout<<"how many testcase do you want? ";
cin>>n;
}
void straight_line::show()
{
int x,y,i,rem=0,quo=0,temp=0,arr[n];
cout<<"Enter the value of x and y: ";
for(i=1; i<=n; i++)
{
cin>>x>>y;
cout<<endl;
if(x<y)
{
temp=y-x-2;
rem=temp%2;
quo=temp/2;
arr[i]=rem+quo+2;
}
}
cout<<"result: "<<endl;
for(i=1; i<=n; i++)
cout<<arr[i]<<endl;
}
main()
{
straight_line obj;
obj.show();
}
PROBLEM 1::SOLUTION 2
ReplyDelete#include
using namespace std;
class straight_line
{
public:
int x,y,n,i,arr[1000];
straight_line(int t);
~straight_line();
void show();
};
straight_line::straight_line(int t)
{
n=t;
int rem=0,quo=0,temp=0;
cout<<"Enter the value of x and y: ";
for(i=1; i<=n; i++)
{
cin>>x>>y;
cout<>testcase;
straight_line obj(testcase);
obj.show();
}
#include
ReplyDeleteusing namespace std;
class Box
{
public:
double l, h , b;
double volume()
{
return (l * h * b);
}
Box(double a, double b, double c)
{
l = a;
h = b;
b = c;
}
Box();
~Box()
{
cout <<"Des activate"<< endl;
}
};
int main()
{
double i, j, k;
cout <<"Enter the l of box1: ";
cin >> i;
cout<<"Enter the h of box1: ";
cin>>j;
cout<<"Enter the b of box1: ";
cin>>k;
Box box1(i,j,k);
cout<<"Enter the l of box2: ";
cin>>i;
cout<<"Enter the h of box2: ";
cin>>j;
cout<<"Enter the b of box2: ";
cin>>k;
Box box2(i,j,k);
cout <<"The volume of box1: "<< box1.volume() <<endl;
cout<<"The volume of box2: "<< box2.volume() <<endl;
return 0;
}
Maisha Fahmida
Batch:48th
ID:201511018
#include
ReplyDeleteusing namespace std;
class rec
{
int h;
int w;
public:
void set(int h, int w);
int area();
rec();
~rec();
};
int rec::area()
{
return h * w;
}
rec::rec()
{
h=10;
w=12;
cout<<"const"<<endl;
}
rec::~rec()
{
cout<<"Dest"<<endl;
}
int main()
{
rec obj;
cout<<"area="<<obj.area()<<endl;
return 0;
}
Maisha Fahmida
Batch:48th
ID:201511018